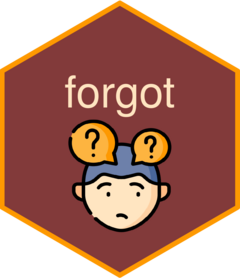
Print examples for an R function of interest in console
forgot_exmpls.Rd
Print examples for an R function of interest in console
Examples
forgot_exmpls("dplyr", "count")
#>
#> # count() is a convenient way to get a sense of the distribution of
#> # values in a dataset
#> starwars %>% count(species)
#> starwars %>% count(species, sort = TRUE)
#> starwars %>% count(sex, gender, sort = TRUE)
#> starwars %>% count(birth_decade = round(birth_year, -1))
#>
#> # use the `wt` argument to perform a weighted count. This is useful
#> # when the data has already been aggregated once
#> df <- tribble(
#> ~name, ~gender, ~runs,
#> "Max", "male", 10,
#> "Sandra", "female", 1,
#> "Susan", "female", 4
#> )
#> # counts rows:
#> df %>% count(gender)
#> # counts runs:
#> df %>% count(gender, wt = runs)
#>
#> # When factors are involved, `.drop = FALSE` can be used to retain factor
#> # levels that don't appear in the data
#> df2 <- tibble(
#> id = 1:5,
#> type = factor(c("a", "c", "a", NA, "a"), levels = c("a", "b", "c"))
#> )
#> df2 %>% count(type)
#> df2 %>% count(type, .drop = FALSE)
#>
#> # Or, using `group_by()`:
#> df2 %>% group_by(type, .drop = FALSE) %>% count()
#>
#> # tally() is a lower-level function that assumes you've done the grouping
#> starwars %>% tally()
#> starwars %>% group_by(species) %>% tally()
#>
#> # both count() and tally() have add_ variants that work like
#> # mutate() instead of summarise
#> df %>% add_count(gender, wt = runs)
#> df %>% add_tally(wt = runs)
forgot_exmpls(dplyr, count, write = FALSE)
#>
#> # count() is a convenient way to get a sense of the distribution of
#> # values in a dataset
#> starwars %>% count(species)
#> starwars %>% count(species, sort = TRUE)
#> starwars %>% count(sex, gender, sort = TRUE)
#> starwars %>% count(birth_decade = round(birth_year, -1))
#>
#> # use the `wt` argument to perform a weighted count. This is useful
#> # when the data has already been aggregated once
#> df <- tribble(
#> ~name, ~gender, ~runs,
#> "Max", "male", 10,
#> "Sandra", "female", 1,
#> "Susan", "female", 4
#> )
#> # counts rows:
#> df %>% count(gender)
#> # counts runs:
#> df %>% count(gender, wt = runs)
#>
#> # When factors are involved, `.drop = FALSE` can be used to retain factor
#> # levels that don't appear in the data
#> df2 <- tibble(
#> id = 1:5,
#> type = factor(c("a", "c", "a", NA, "a"), levels = c("a", "b", "c"))
#> )
#> df2 %>% count(type)
#> df2 %>% count(type, .drop = FALSE)
#>
#> # Or, using `group_by()`:
#> df2 %>% group_by(type, .drop = FALSE) %>% count()
#>
#> # tally() is a lower-level function that assumes you've done the grouping
#> starwars %>% tally()
#> starwars %>% group_by(species) %>% tally()
#>
#> # both count() and tally() have add_ variants that work like
#> # mutate() instead of summarise
#> df %>% add_count(gender, wt = runs)
#> df %>% add_tally(wt = runs)
if (FALSE) { # \dontrun{
forgot_exmpls("dplyr", "count", write = TRUE)
} # }